AlertDialog
は、ユーザーにメッセージを表示し、ユーザーの操作を促すダイアログを作成するために使われます。
このページでは。AlertDialog
の基本的な使い方について解説します。
目次
AlertDialogの書き方
AlertDialog(
onDismissRequest = { /* 画面の外側がタップされた時の処理 */ },
title = { /* タイトル */ },
text = { /* メッセージの内容 */ },
confirmButton = { /* 確認ボタン(「OK」や「はい」など) */ },
dismissButton = { /* 画面を閉じるボタン(「キャンセル」や「閉じる」など)*/ }
)
以下、簡単なダイアログのレイアウト例です。
@Composable
fun MyDialog() {
AlertDialog(
onDismissRequest = {
//画面の外側がタップされた時の処理
},
title = { Text(text = "削除確認") },
text = {
Text(text = "削除しますか")
},
confirmButton = {
TextButton(onClick = { /*TODO*/ }) {
Text(text = "OK")
}
},
dismissButton = {
TextButton(onClick = { /*TODO*/ }) {
Text(text = "キャンセル")
}
}
)
}
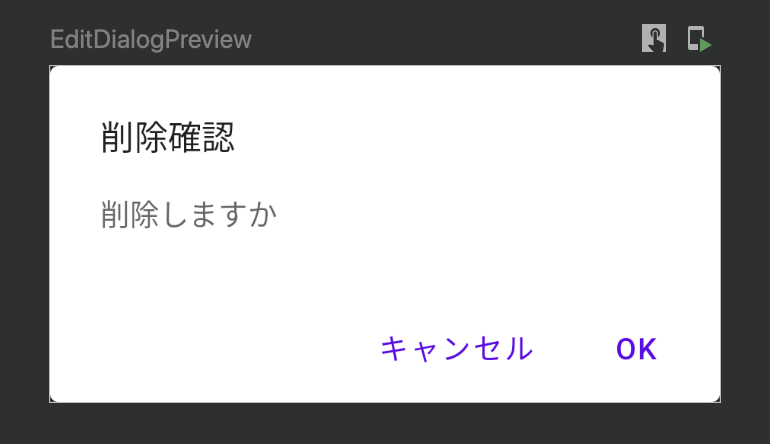
ボタンが押されたらダイアログを表示する
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material.*
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import com.naoyaono.jetpack_compose_ui.ui.theme.Jetpack_Compose_UITheme
@Composable
fun AlertDialogSample() {
// AlertDialogを表示するための状態を定義します
var showDialog by remember { mutableStateOf(false) }
// AlertDialogを表示するトリガーとなるボタンを作成します
Column(
modifier = Modifier.fillMaxSize(),
verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Button(
onClick = { showDialog = true }
) {
Text(text = "アラートダイアログを表示")
}
}
// ボタンが押されたらダイアログを表示
if (showDialog)
AlertDialog(
onDismissRequest = {
//画面の外側がタップされたらダイアログを閉じる
showDialog = false
},
title = { Text(text = "削除確認") },
text = { Text(text = "削除しますか") },
confirmButton = {
TextButton(
onClick = { showDialog = false }
) {
Text(text = "OK")
}
},
dismissButton = {
TextButton(
onClick = { showDialog = false }
) {
Text(text = "キャンセル")
}
}
)
}
@Preview(showBackground = true)
@Composable
fun AlertDialogSamplePreview() {
Jetpack_Compose_UITheme() {
AlertDialogSample()
}
}
この例では、画面中央に配置したボタンがタップされると showDialog
変数が true
になり、AlertDialog が表示されます。
AlertDialog の中にはび確認ボタンやキャンセルボタンがあり、それらをタップされるとshowDialog
変数が false
になるのでダイアログが非表示に、つまりダイアログが閉じられます。
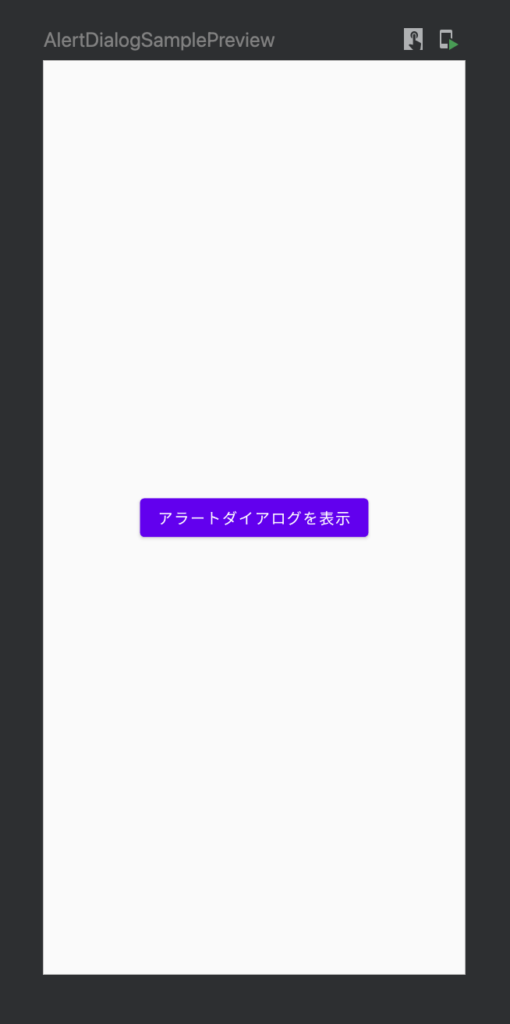
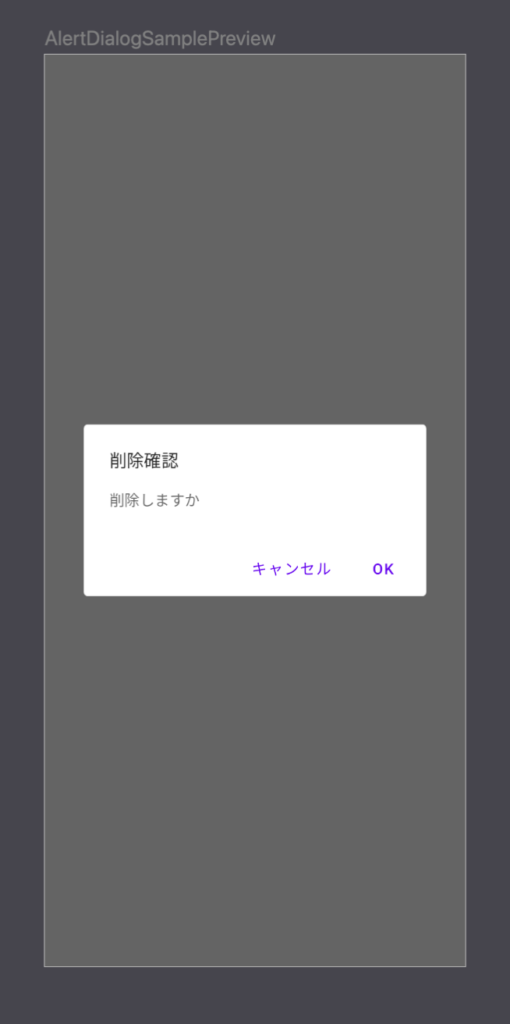
Comment